ScifeonConsoleLogger
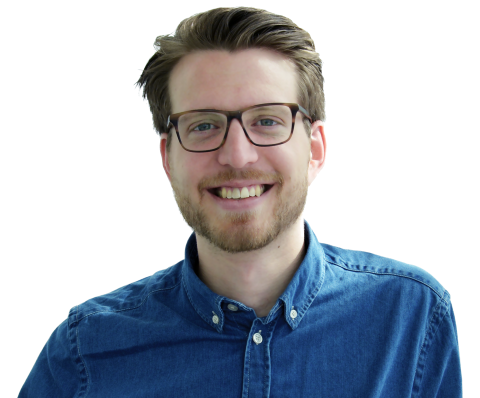
- Example
- API
constructor(app: any, loggerName: string): ScifeonConsoleLogger
logLevel: [LogLevel](loglevel)
setLevel(logLevel: [LogLevel](loglevel)): void
debug(message: string, ...rest: any[]): void
info(message: string, ...rest: any[]): void
warn(message: string, ...rest: any[]): void
error(message: string, ...rest: any[]): void
This class is used for logging to the console from pages, data loaders, etc.
Example
import { inject, ConsoleLogger } from 'scifeon';
@inject(ConsoleLogger)
export class CustomPage {
constructor(logger) {
logger.debug('The logger can now be used.');
logger.setLevel(logger.logLevel.info);
logger.debug('This message will not be shown, as the log level hides it.');
}
}
Typically you would want to set the logger
on the object (this
) using this.logger = logger
.
API
constructor(app: any, loggerName: string): ScifeonConsoleLogger
constructor(app: any, loggerName: string): ScifeonConsoleLogger
app
is the contributions.json
parsed as a Javascript-object. The loggerName
should be something meaningful, e.g. 'page.customPageId'. When the ScifeonConsoleLogger
is injected using dependency injection, these values are automatically set.
logLevel: LogLevel
logLevel: LogLevel
This is a constant with the values defined in LogLevel
.
setLevel(logLevel: LogLevel): void
setLevel(logLevel: LogLevel): void
Sets the level of logging for this logger instance.
Parameters
logLevel
Matches a value of logLevel specifying the level of logging.
debug(message: string, ...rest: any[]): void
debug(message: string, ...rest: any[]): void
Logs a debug message.
Parameters
message: string
The message to log....rest: any[]
The data to log.
Example
import { inject, ConsoleLogger } from 'scifeon';
@inject(ConsoleLogger)
export class CustomPage {
constructor(logger) {
logger.setLevel(logger.logLevel.debug);
logger.debug('The logger can now be used.');
const obj = { extraInfo: 'info' };
logger.debug('The rest parameter can be used to log extra information about', obj, ' and the logger itself', logger);
logger.debug('In the console you will we an array with these extra entries.');
}
}
info(message: string, ...rest: any[]): void
info(message: string, ...rest: any[]): void
Logs info.
Parameters
message: string
The message to log....rest: any[]
The data to log.
warn(message: string, ...rest: any[]): void
warn(message: string, ...rest: any[]): void
Logs a warning.
Parameters
message: string
The message to log....rest: any[]
The data to log.
error(message: string, ...rest: any[]): void
error(message: string, ...rest: any[]): void
Logs an error.
Parameters
message: string
The message to log....rest: any[]
The data to log.