ScifeonDialog
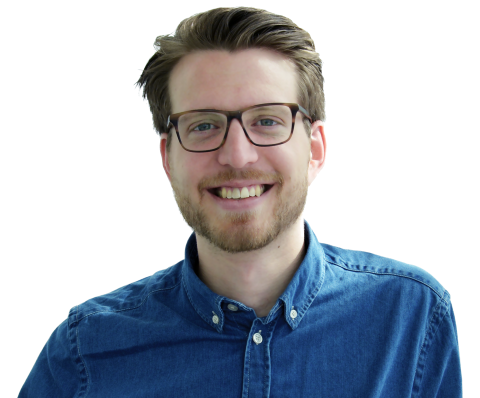
- Example
- API
- Methods
open(options: any): DialogOpenPromise<DialogOpenResult | DialogCancelResult>
confirm(question: string): Promise<boolean>
message(message: string): DialogOpenPromise<DialogOpenResult | DialogCancelResult>
close(ok: boolean = true, object: any = null): void
cancel(output: any = null): void
ok(output: any = null): void
- Methods
This class is used for showing dialogs (or popups). The class is wrapping the Aurelia Dialog-plugin, which is why there are some references to the documentation of this.
Example
API
Methods
open(options: any): DialogOpenPromise<DialogOpenResult | DialogCancelResult>
open(options: any): DialogOpenPromise<DialogOpenResult | DialogCancelResult>
Parameters
options
An object with the following possible values:options = { lock: boolean, // If false, the dialog can be closes by pressing ESC or clicking the overlay model: any, // An object passed to the activate() method of the view model viewModel: any, // The view model view: string, // A HTML string, typically just the view corresponding to the view model style: string, // A CSS string, e.g. 'width: 800px;' header: string, // A HTML string footer: string // A HTML string - defaults to an OK and Cancel button };
Returns
A DialogClosePromise
, with the method .whenClosed(result => ...)
.
Example
import { MyDialogViewModel } from './my-dialog-view-model';
...
openDialog() {
this.dialog.open({
viewModel: MyDialogViewModel,
view: require('./my-dialog-view-model.html')
}).whenClosed(result => /* do something with the result */);
}
...
confirm(question: string): Promise<boolean>
confirm(question: string): Promise<boolean>
Show a simple popup with the question and 'Yes' and 'No' buttons.
Parameters
question
The question to show.
Returns
Returns true or false through a promise.
Example
...
async openConfirmDialog() {
const result = await this.dialog.confirm('Yes or no?');
if (result) {
// do something when Yes is pressed
} else {
// do something else when No is pressed
}
}
...
message(message: string): DialogOpenPromise<DialogOpenResult | DialogCancelResult>
message(message: string): DialogOpenPromise<DialogOpenResult | DialogCancelResult>
Show a simple popup with the message and an 'OK' button.
Parameters
message
The message to show.
Returns
Returns a promise with a true value.
Example
...
async openMessageDialog() {
const result = await this.dialog.message('A message');
if (result) {
// do something when the message has been shown
}
}
...
close(ok: boolean = true, object: any = null): void
close(ok: boolean = true, object: any = null): void
Closes the current dialog.
Parameters
ok
Whether the result should be ok or not.object
The object to return to the callee of the current dialog.
cancel(output: any = null): void
cancel(output: any = null): void
Cancels (and closes) the current dialog.
Parameters
object
The object to return to the callee of the current dialog.
ok(output: any = null): void
ok(output: any = null): void
Closes the current dialog with an OK value.
Parameters
object
The object to return to the callee of the current dialog.