ScifeonUser
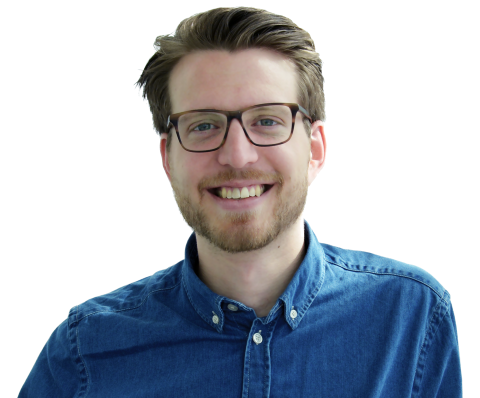
This class contains information about the currently logged on user.
Example
import { User } from 'scifeon';
export class CustomPage {
constructor() {
if (User.isAdmin) {
// do something when admin
}
this.userId = User.id;
}
}
API
Methods
static get(): ScifeonUser
static get(): ScifeonUser
Returns
The currently logged in user.
Properties
static id: string
static id: string
The database id of the currently logged on user.
static email: string
static email: string
The email of the currently logged on user.
static displayName: string
static displayName: string
static firstName: string
static firstName: string
static middleNames: string
static middleNames: string
static lastName: string
static lastName: string
static phone: string
static phone: string
static departmentID: string
static departmentID: string
The department id of the currently logged on user.
static isActive: boolean
static isActive: boolean
True if the currently logged on user is active. This will always be true, as the user cannot log in if it inactive.
static isAdmin: boolean
static isAdmin: boolean
True if the currently logged on user is system administrator.
static Helper: ScifeonUserHelper
static Helper: ScifeonUserHelper
An instantiated instance of the ScifeonUserHelper
class.
Constants
ROLE_KEYS: Map<string, string>
ROLE_KEYS: Map<string, string>
A map of allowed roles, currently:
ADMIN
Example
This can be used in conjunction with hasOneOfRoles()
:
import { User } from 'scifeon';
export class TestClass {
constructor() {
if (User.hasOneOfRoles(User.ROLE_KEYS.ADMIN)) {
this.adminMessage = 'Is admin';
}
}
}